Need some help with my form validation please!
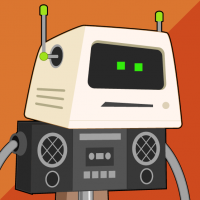
in Off-Topic
I wrote this form and I am having trouble figuring out how to do 1 of either two things:
1.) Display all missing fields in red on the same window (i tried using document.write but no success)
2.) Display all missing fields in an alert box (using alert()...)
Right now, for each missing field there is an alert(). Can anybody offer some advice or help me figure this out? My current functional code is below.
Many thanks in advance!!!
<SCRIPT>
//--> Begin
function validate()
{
// Check age group dropdown
if (mainform.age.options[0].selected)
{alert('Please select your age group.');
event.returnValue=false;}
// Check referral dropdown
if (mainform.referred.options[0].selected)
{alert('How did you hear about AnthonyJD.com?');
event.returnValue=false;}
// If referral dropdown is Other, make sure its specified where
mr2v=mainform.reason2.value;
if (mainform.referred.options[4].selected && mr2v=='')
{alert('How did you hear about AnthonyJD.com?');
event.returnValue=false;}
// Check enjoy website radio buttons
if (!(mainform.enjoysite[0].checked || mainform.enjoysite[1].checked))
{alert('Have you enjoyed browsing AnthonyJD.com?');
event.returnValue=false;}
// If radio button is No, make sure reason is filled in
mrv=mainform.reason.value;
if (mrv=='' && mainform.enjoysite[1].checked)
{alert('What did you not like about AnthonyJD.com?');
event.returnValue=false;}
// Check visit again dropdown
if (mainform.visitagain.options[0].selected)
{alert('Are you likely to visit AnthonyJD.com again?');
event.returnValue=false;}
}
//--> End
</SCRIPT>
1.) Display all missing fields in red on the same window (i tried using document.write but no success)
2.) Display all missing fields in an alert box (using alert()...)
Right now, for each missing field there is an alert(). Can anybody offer some advice or help me figure this out? My current functional code is below.
Many thanks in advance!!!

<SCRIPT>
//--> Begin
function validate()
{
// Check age group dropdown
if (mainform.age.options[0].selected)
{alert('Please select your age group.');
event.returnValue=false;}
// Check referral dropdown
if (mainform.referred.options[0].selected)
{alert('How did you hear about AnthonyJD.com?');
event.returnValue=false;}
// If referral dropdown is Other, make sure its specified where
mr2v=mainform.reason2.value;
if (mainform.referred.options[4].selected && mr2v=='')
{alert('How did you hear about AnthonyJD.com?');
event.returnValue=false;}
// Check enjoy website radio buttons
if (!(mainform.enjoysite[0].checked || mainform.enjoysite[1].checked))
{alert('Have you enjoyed browsing AnthonyJD.com?');
event.returnValue=false;}
// If radio button is No, make sure reason is filled in
mrv=mainform.reason.value;
if (mrv=='' && mainform.enjoysite[1].checked)
{alert('What did you not like about AnthonyJD.com?');
event.returnValue=false;}
// Check visit again dropdown
if (mainform.visitagain.options[0].selected)
{alert('Are you likely to visit AnthonyJD.com again?');
event.returnValue=false;}
}
//--> End
</SCRIPT>
Comments
-
AnthonyJD81 Member Posts: 187
I was able to figure out a way to do this. Here is the script for those who are interested at all...I figure I might as well share the knowledge
var error;
var error_a;
var error_r;
var error_e;
var error_v;
var errormsg;
function reset()
{
error_a=false;
error_r=false;
error_e=false;
error_v=false;
errormsg='The following fields were missing :\n_____________________________\n\n';
}
//*************************************************************************
// Check to make sure age dropdown box is selected.
//*************************************************************************
function validate_age()
{
if (mainform.age.options[0].selected)
{
errormsg+='Please select your age\n';
error_a=true;
}
}
//*************************************************************************
// Check to make sure referred dropdown box is selected.
// If Other, make sure reason is specified in the text box
//*************************************************************************
function validate_refer()
{
if (mainform.referred.options[0].selected)
{
errormsg+='How did you hear about AnthonyJD.com?\n';
error_r=true;
}
if (mainform.referred.options[4].selected && mainform.reason2.value=='')
{
errormsg+='How did you hear about AnthonyJD.com?\n';
error_r=true;
}
}
//*************************************************************************
// Check to make sure enjoysite radio button is selected.
// If No, make sure reason is specified in the text box
//*************************************************************************
function validate_enjoy()
{
if (mainform.reason.value=='' && mainform.enjoysite[1].checked)
{
errormsg+='What did you not like about AnthonyJD.com?\n';
error_e=true;
}
if (!(mainform.enjoysite[0].checked || mainform.enjoysite[1].checked))
{
errormsg+='Did you enjoy this site?\n';
error_e=true;
}
}
//*************************************************************************
// Check to make sure visit again dropdown box is selected.
//*************************************************************************
function validate_visit()
{
if (mainform.visitagain.options[0].selected)
{
errormsg+='Will you visit again AnthonyJD.com again?\n';
error_v=true;
}
}
//*************************************************************************
// Begin Validation
//*************************************************************************
function validate()
{
reset();
validate_age();
validate_refer();
validate_enjoy();
validate_visit();
if(error_a||error_r||error_e||error_v)
{
error=true;
}
else
{
error=false;
}
if(!error)
{
document.main.submit();
}
else
{
alert(errormsg);
event.returnValue=false;
}
}
I just need to test it out with NN, Opera, and Mozilla now and work out any bugs or problems... -
Webmaster Admin Posts: 10,292 Admin
Nice... I don't understand it entirely (I'm a lauzy developer) but it looks like a clean solution.
I just need to test it out with NN, Opera, and Mozilla now
Why? -
AnthonyJD81 Member Posts: 187
The script is actually quite simple when you think about it...you have a form. On submit, function Validate() runs. Within Validate() is another set of functions. Each of these sub functions is one of the questions on my contact form. It runs through each one and makes sure they are required. If one isn't filled in (or false) the error message get stored and at the end alerted. If all the answers are filled in (or true) then the form gets submitted....help at all
Why those four browsers you ask?
...because I would really like to cater to everyone I possibly can.
So you ask why those four in particular? For starters, I have all four installed on my machine and I am able to test them. Second I like them, well with the exception for NN
Besides, aren't these four of the most popular? I mean everywhere I look, developers are ranting and raving about how Opera this, Netscape that, etc....I figured I would tackle building a site that these browsers could see perfectly.
My first site I had up was IE perfect. Pretty much everything else was choppy in some form or another. I took that site down and I am in the process of building another one now. My new layout consists mainly of CSS and of course some JS and HTML here and there. And of course some of these browsers are not complaint with W3C's structure...but oh well. Just makes it harder for me i guess
Eventually, I will begin learning PHP and maybe .NET but on the flipside, I need to earn some more certs...like my CCNA and MCSA
I will post a link to the form for you to see first hand once I load it up. -
Webmaster Admin Posts: 10,292 Admin
The stats for TechExams.net over the last 8 months (over 350,000 visitors):
IE: 97,3%
NN: 1.9%
Others: 0.8%
But if you have all the browsers installed, why not test it... I wouldn't go through to much hassle if it doesn't work on any of the 'Others' though. I actually stopped testing my sites on other browsers years ago, 50% of creating a site went into making it work for 5% Netscape users...
I usually don't have feelings for software but I really dislike Netscape, as soon as I install it I get: -
AnthonyJD81 Member Posts: 187
Webmaster wrote:The stats for TechExams.net over the last 8 months (over 350,000 visitors):
IE: 97,3%
NN: 1.9%
Others: 0.8%
Wow, pretty amazing. I thought the stats would be more spread. Very interesting. Thanks for pointing that out to me. How did you gather these results? Through a poll? I haven't seen one on here. I must have missed it if you did...sorryWebmaster wrote:But if you have all the browsers installed, why not test it... I wouldn't go through to much hassle if it doesn't work on any of the 'Others' though. I actually stopped testing my sites on other browsers years ago, 50% of creating a site went into making it work for 5% Netscape users...
I usually don't have feelings for software but I really dislike Netscape, as soon as I install it I get:
I wasn't planning on spending to much time "working" for the other browsers. Thanks for pointing some of that usefuly info out to me. I really appreciate it -
Webmaster Admin Posts: 10,292 Admin
P42GDell wrote:How did you gather these results? Through a poll? I haven't seen one on here. I must have missed it if you did...sorry
These are from the site's stats gathered by www.Hitboxcentral.com, that is why the small banner is on the bottom of almost every page. -
AnthonyJD81 Member Posts: 187
I looked into HitBob but didn't want to shell out the money for it right now, although it looks very nice
I went with Extreme Tracking -> http://www.extreme-dm.com/tracking/
Here is my site, http://www.anthonyjd.com and you can see the statistics since I put it up last night...82% are all IE users...i like how this service breaks down everything to the dime....pretty impressive -
Webmaster Admin Posts: 10,292 Admin
hitboxcentral is free, like I said that is why the banner is on the bottom of most pages at TechExams.net. It's a lot bigger than the one you're using but the stats at hitboxcentral easily make up for that... well at least in my case.