Java storage - arraylist?
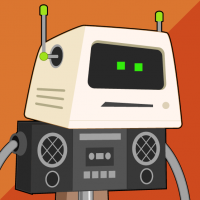
Hi Guys,
Im a total newbie at java so excuse the crap terminology i'll probably use. Anyway, im working on a client/server task for a module im studying which is similar to a kind of inventory. basically the client has options on the interface and the server interface displays the inventory - its ID, description etc etc. Now so far the server inventory data has been statically defined in the server class. However i want to ammend it so i can add an additional option to the client interface to add new items to the inventory.
Would i be right in thinking an arraylist is the best thing to store the information input from the user?
im starting to get a little stumped because we've never had a java lecture or anything to do with it before but where going to be getting coursework on it!
...without having any lectures on the coding!!!
..no idea where that logic comes from
Im a total newbie at java so excuse the crap terminology i'll probably use. Anyway, im working on a client/server task for a module im studying which is similar to a kind of inventory. basically the client has options on the interface and the server interface displays the inventory - its ID, description etc etc. Now so far the server inventory data has been statically defined in the server class. However i want to ammend it so i can add an additional option to the client interface to add new items to the inventory.
Would i be right in thinking an arraylist is the best thing to store the information input from the user?
im starting to get a little stumped because we've never had a java lecture or anything to do with it before but where going to be getting coursework on it!

..no idea where that logic comes from

Xbox Live: Bring It On
Bsc (hons) Network Computing - 1st Class
WIP: Msc advanced networking
Bsc (hons) Network Computing - 1st Class
WIP: Msc advanced networking
Comments
-
JDMurray Admin Posts: 13,090 Admin
An ArrayList is for storing related information that is all the same data type. For example, if you have the ages of 100 people you could store them in an integer ArrayList 100 elements in size.
If you can't modify the original class storing the inventory information, you'll need to write a new class to extend the original class, such as:public class InventoryInfo { public int ID; public string Name; public string Description; }; public class InventoryInfo2 extends InventoryInfo { public Date LastModified; public long PartNumber; };
The InventoryInfo class contains the original information, and InventoryInfo2 contains everything in InventoryInfo plus the new fields. -
nel Member Posts: 2,859 ■□□□□□□□□□
ahh, so you could not store both strings and integers. So would a hashtable be more what im looking for? im trying to store the information input by the client which will include both int and strings.Xbox Live: Bring It On
Bsc (hons) Network Computing - 1st Class
WIP: Msc advanced networking -
JDMurray Admin Posts: 13,090 Admin
nel wrote:ahh, so you could not store both strings and integers. So would a hashtable be more what im looking for? im trying to store the information input by the client which will include both int and strings.public class InventoryInfo { public int ID; public string Name; public string Description; InventoryInfo(id, name, desc) { ID = id; Name = name; Description = desc; } }; ArrayList<InventoryInfo> invenArray = new ArrayList<InventoryInfo>(); invenArray.add(new InventoryInfo("001", "Nuts", "Hardware")); invenArray.add(new InventoryInfo("002", "Bolts", "Hardware")); invenArray.add(new InventoryInfo("003", "Wrench", "Tool"));
-
nel Member Posts: 2,859 ■□□□□□□□□□
Ah, Thanks for that JD.
Sorry for being stupid!Xbox Live: Bring It On
Bsc (hons) Network Computing - 1st Class
WIP: Msc advanced networking