help with program
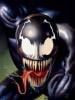
in Off-Topic
can someone help me with my program? I am using C++ and trying to create objects that contain a vector and an initial size of the vector. I am not implementing the constructor correctly because as soon as my object leaves the constructor its size gets reset to 0. Could someone please help me out so that when I use the insertElement function it keeps the objects size.
#include <iostream>
#include <vector>
using std::cout;
using std::cin;
using std::endl;
using std::vector;
class List
{
public:
List(int=10);
void insertElement(int); //first int will represent value second will represent position
private:
vector <int> vect;
int size;
};
List::List(int listsize)
{
size=listsize; //assigning inputed integer in main to data member size
vector<int> vect(size);
for(int i=0; i<vect.size(); i++)
vect= i+1;
cout << vect.size() << endl;
}
void List::insertElement(int value)
{
vect.push_back(value);
cout << vect.size() << endl;
}
int main()
{
List list1(9);
list1.insertElement(44);
list1.insertElement(33);
return 0;
}
#include <iostream>
#include <vector>
using std::cout;
using std::cin;
using std::endl;
using std::vector;
class List
{
public:
List(int=10);
void insertElement(int); //first int will represent value second will represent position
private:
vector <int> vect;
int size;
};
List::List(int listsize)
{
size=listsize; //assigning inputed integer in main to data member size
vector<int> vect(size);
for(int i=0; i<vect.size(); i++)
vect= i+1;
cout << vect.size() << endl;
}
void List::insertElement(int value)
{
vect.push_back(value);
cout << vect.size() << endl;
}
int main()
{
List list1(9);
list1.insertElement(44);
list1.insertElement(33);
return 0;
}
Comments
-
2lazybutsmart Member Posts: 1,119
when ur program executes this line:
cout << vect.size() << endl;
does size contain the value u want? because ur constructor looks ok except for that 10 ur initilizing in the prototype. the only way size could loose it's data when the program exits the constructor part is that the vector stuff changes the values. and ur not using size as a static datamember throughout many instances of the List class.
so u might want to verify what really happens in the constructor.
2lbs.
p.s. how come ur header files don't have the ".h" in thier declarationsExquisite as a lily, illustrious as a full moon,
Magnanimous as the ocean, persistent as time.