Options
DirectorySearcher filter (PowerShell/C#/ADSI Edit)
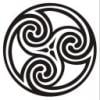
in Off-Topic
Ok, I am trying to build a filter dynamically in C# using DirectorySearcher and I am having some issues. Things to keep in mind:
1. We are in a multi-forest, multi-domain AD environment.
2. There are two main forests company.net and jointVenture.net
3. Company.net has multiple domains.
4. Most of my users will have accounts in company.net.
5. Everything is authenticating against jointVesture.net's infrastructure.
All I have is the string DOMAIN\UserName. How do I build the filter?
((sAMAccount=rkaucher)(DC=company)(DC=net)) but this does not return anything...
Here is my code as it stands right now:
I will probably cross post this on StackOverflow. But since the same syntax can be used in PoSh I figured I'd give it a go here first.
DirectorySearcher Class (System.DirectoryServices)
LDAP Dialect (Windows)
Search Filter Syntax (Windows)
1. We are in a multi-forest, multi-domain AD environment.
2. There are two main forests company.net and jointVenture.net
3. Company.net has multiple domains.
4. Most of my users will have accounts in company.net.
5. Everything is authenticating against jointVesture.net's infrastructure.
All I have is the string DOMAIN\UserName. How do I build the filter?
((sAMAccount=rkaucher)(DC=company)(DC=net)) but this does not return anything...
Here is my code as it stands right now:
protected override User GetAuthenticatedUser(System.Security.Principal.IPrincipal principal) { User user = new User(); user.Name = principal.Identity.Name; DirectorySearcher dsr = new DirectorySearcher(); DirectoryEntry der = new DirectoryEntry(); if (principal.Identity.Name.Split('\\')[0] == "AM") { dsr.Filter += "((sAMAccountName=" + principal.Identity.Name.Split('\\')[1]+")"; dsr.Filter += "(DC=" + principal.Identity.Name.Split('\\')[0]; dsr.Filter += ")(DC=company)(DC=net))"; } else if(principal.Identity.Name.Split('\\')[0] == "jointVenture") { dsr.Filter = principal.Identity.Name.Split('\\')[1]; } DirectoryEntry result = dsr.FindOne().GetDirectoryEntry(); user.FullName = Convert.ToString(result.Properties["displayName"].Value); return user; } }
I will probably cross post this on StackOverflow. But since the same syntax can be used in PoSh I figured I'd give it a go here first.
DirectorySearcher Class (System.DirectoryServices)
LDAP Dialect (Windows)
Search Filter Syntax (Windows)
Comments
-
Options
RobertKaucher Member Posts: 4,299 ■■■■■■■■■■
protected override User GetAuthenticatedUser(System.Security.Principal.IPrincipal principal) { User user = new User(); user.Name = principal.Identity.Name; DirectorySearcher dsr = new DirectorySearcher(); dsr.Filter = "sAMAccountName=" + principal.Identity.Name.Split('\\')[1]; if (principal.Identity.Name.Split('\\')[0] != "jointVenture") { dsr.SearchRoot = new DirectoryEntry("[URL="ldap://dc="+principal.Identity.Name.Split('\\')[0]+",DC=company,dc=net"]LDAP://dc="+principal.Identity.Name.Split('\\')[0]+",DC=company,dc=net[/URL]"); } else if(principal.Identity.Name.Split('\\')[0] == "jointVenture") { dsr.SearchRoot = new DirectoryEntry("[URL]ldap://dc=jointVenture,dc=net[/URL]"); } DirectoryEntry result = dsr.FindOne().GetDirectoryEntry(); user.FullName = Convert.ToString(result.Properties["displayName"].Value); return user; } }
A coworker and I figured it out. Two eyes.