Powershell Issue
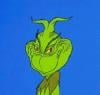
in Off-Topic
One of our developers came to met today asking if I could right a script to backup a config file to another folder on the server. He added an "extra credit" if I could get it to make sure no more that 14 backup files were in there. Of course I jumped to powershell and I actually wrote the script correctly, but there's a slight issue. Basically, here is what it is suppose to do:
1. Get a count of the number of files in ISAPI_Backup and assign that value to $count
2. If $count is less then 14, copy httpd.conf to ISAPI_Backup and append the date to the name
3. If $count is greater then 14, delete the oldest backup file
4. Then copy httpd.conf to ISAPI_Backup and append the date to the name
That all works, except each time it runs it appends the date to all the files in the folder and so we ran three tests...it appended the date to all the files in each run. Any ideas? Code is below.
$count = (Get-ChildItem "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" -recurse | where-object {-not ($_.PSIsContainer)}).Count
Write-Host $count
IF ($count -lt 14) {
copy-item "C:\Program Files\Helicon\ISAPI_Rewrite3\httpd.conf" "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup"
dir "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" | Rename-Item -NewName {$_.BaseName+(Get-Date -f yyyy-MM-dd)+$_.Extension}}
ELSEIF ($count -gt 14) {
Get-ChildItem "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" | Sort CreationTime | Select -First 1 | Remove-Item}
ELSE {copy-item "C:\Program Files\Helicon\ISAPI_Rewrite3\httpd.conf" "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup"
dir "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup\httpd.conf" | Rename-Item -NewName {$_.BaseName+(Get-Date -f yyyy-MM-dd)+$_.Extension}}
1. Get a count of the number of files in ISAPI_Backup and assign that value to $count
2. If $count is less then 14, copy httpd.conf to ISAPI_Backup and append the date to the name
3. If $count is greater then 14, delete the oldest backup file
4. Then copy httpd.conf to ISAPI_Backup and append the date to the name
That all works, except each time it runs it appends the date to all the files in the folder and so we ran three tests...it appended the date to all the files in each run. Any ideas? Code is below.
$count = (Get-ChildItem "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" -recurse | where-object {-not ($_.PSIsContainer)}).Count
Write-Host $count
IF ($count -lt 14) {
copy-item "C:\Program Files\Helicon\ISAPI_Rewrite3\httpd.conf" "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup"
dir "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" | Rename-Item -NewName {$_.BaseName+(Get-Date -f yyyy-MM-dd)+$_.Extension}}
ELSEIF ($count -gt 14) {
Get-ChildItem "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" | Sort CreationTime | Select -First 1 | Remove-Item}
ELSE {copy-item "C:\Program Files\Helicon\ISAPI_Rewrite3\httpd.conf" "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup"
dir "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup\httpd.conf" | Rename-Item -NewName {$_.BaseName+(Get-Date -f yyyy-MM-dd)+$_.Extension}}
WIP:
PHP
Kotlin
Intro to Discrete Math
Programming Languages
Work stuff
PHP
Kotlin
Intro to Discrete Math
Programming Languages
Work stuff
Comments
-
ptilsen Member Posts: 2,835 ■■■■■■■■■■
I'm not sure I understand the problem correctly. Is the issue that it re-appends the date to each backup file on each subsequent run, rather than not renaming the files?
The code you have will rename everything found in C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup every time there are fewer than 14 files in the directory.
Is there a reason you're appending it in this manner? I would do the append as part of the copy:
$date = Get-Date -f yyyy-MM-dd
$dstFileName = "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup\httpd$date.conf
copy-item "C:\Program Files\Helicon\ISAPI_Rewrite3\httpd.conf" $dstFileName
Also, if I read this correctly, it will not backup the file if count is greater than 14 at all. Your requirements indicate it should copy it regardless of the count, but there is no copy statement under the ELSEIF. -
the_Grinch Member Posts: 4,165 ■■■■■■■■■■
My bad, should explain it better. Basically it is doing the following:
Places httpd.conf in the folder, with the date appended. At the same time, all of the httpd2013-13-15.confs now look like this
httpd2013-13-152013-13-15.conf and with each run it puts another one in there.
As for appending in that matter, only did it that way because I believed I had to copy it first then rename (thanks for pointing out I could do with with the copy!).
I thought by putting the ELSE after the ELSEIF it acted as a then. Somewhat like the IF...ELSEIF...THEN of VBScript. Is that not the case?
Any help to correctly write this would be awesome and thanks for everything thus far!WIP:
PHP
Kotlin
Intro to Discrete Math
Programming Languages
Work stuff -
ptilsen Member Posts: 2,835 ■■■■■■■■■■
The else would be a separate clause in the selection statement in pretty much any language. Your If, Else, and ElseIf statements are all different options, not nested or unrelated. If A, do x; if B instead of A, do y; if neither A nor B, do z.
Your code currently reads:
Print how many files exist in the specified directory.
If that number is lower than 14, copy the httpd.conf file to the backup directory, then append the current date to all files in the backup directory.
If that number is higher than 14 instead of lower than 14, delete the oldest file and do nothing else.
If that number is neither lower nor higher than 14 (effectively, the number is 14), then copy the httpd.conf file to the backup directory and append the current date to all files in the backup directory.
If you replace the dir | rename with the copy, you could bring that completely outside the selection statement and eliminate most of it:
$date = Get-Date -f yyyy-MM-dd
$dstFileName = "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup\httpd$da te.conf
copy-item "C:\Program Files\Helicon\ISAPI_Rewrite3\httpd.conf" $dstFileName
$count = (Get-ChildItem "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" -recurse | where-object {-not ($_.PSIsContainer)}).Count
Write-Host $count
IF ($count -gt 14) {Get-ChildItem "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" | Sort CreationTime | Select -First 1 | Remove-Item}
Unless I misunderstand the goal, this should accomplish everything. It backs the file up no matter (but prompts for overwrite if you already have a backup that day), then deletes the oldest file if there are 15 or more files in the backup directory.
Also, looking back at the code, your only real mistake was leaving the file off the dir command. In the If, you have:
dir "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup" | Rename-Item -NewName {$_.BaseName+(Get-Date -f yyyy-MM-dd)+$_.Extension}
dir "C:\Program Files\Helicon\ISAPI_Rewrite3\ISAPI_Backup\httpd.conf" | Rename-Item -NewName {$_.BaseName+(Get-Date -f yyyy-MM-dd)+$_.Extension}
The former appends date to every file in the dir, the latter just appends date to that file, since it will be the only output of dir. I would still make the name a variable and then use it in copy anyway, but I wanted to point it out. -
the_Grinch Member Posts: 4,165 ■■■■■■■■■■
Thanks man! I'm definitely going to make the changes you provided! Also, thanks for saying that everything (for the most part) looked good. I figured out part of the issue. Initially, I wrote the script on my machine and tested to make sure it worked. We then moved it to the server and proceeded to make adjustments which is where the problems came into play. Regardless, I really appreciate all the help!WIP:
PHP
Kotlin
Intro to Discrete Math
Programming Languages
Work stuff -
N2IT Inactive Imported Users Posts: 7,483 ■■■■■■■■■■
How many AD accounts did you delete when you ran it on the server?
Just kidding nice work on the PS script. I've been banging a few off of AD recently. SCCM is nice but we are unsure about how much participation we are getting from the SCCM server and the clients (WMI). -
the_Grinch Member Posts: 4,165 ■■■■■■■■■■
It's funny you say that. We're running it on a web server and so before moving it to production we ran it on our test site. Unrelated, but all of a sudden the test site stopped working properly. So the web developer had to spend time fixing that before we could test the script.WIP:
PHP
Kotlin
Intro to Discrete Math
Programming Languages
Work stuff